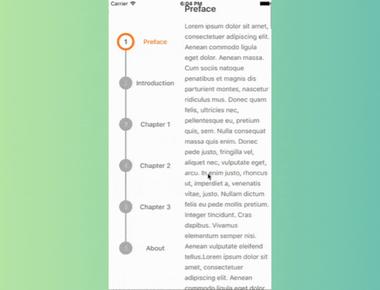
Vertical Step indicator
May 06, 2023
1 min
Animated.Value
between ParallaxSwiper and your own UI.Prop | Type | Default | Description |
---|---|---|---|
speed | Number | 0.25 | This number determines how fast BackgroundComponent moves. Set to 0 for no movement at all, set to 1 and background will move as fast as the scroll. |
dividerWidth | Number | 8 | The width of the divider between each page. (horizontal only) |
dividerColor | String | black | Color of divider. |
backgroundColor | String | black | ParallaxSwiper’s background color. |
scrollToIndex | Number | 0 | Scroll to page with a smooth animation. Note: You need to use state if you want to change index any other time than when component is rendered. |
onMomentumScrollEnd | Function | N/A | Fired when ScrollView stops scrolling and is passed the current page index. |
animatedValue | Number (Animated.Value) | 0 | Optionally pass a new instance of Animated.Value to access the animated value outside of ParallaxSwiper. |
vertical | Boolean | false | When true, ParallaxSwiper’s children are arranged vertically in a column instead of horizontally in a row. For now only iOS supports this. |
showsHorizontalScrollIndicator | Boolean | false | When true, shows a horizontal scroll indicator. The default value is false. |
showsVerticalScrollIndicator | Boolean | false | When true, shows a vertical scroll indicator. The default value is false. |
children | React component (ParallaxSwiperPage) | N/A | Each top-level ParallaxSwiperPage child. |
showProgressBar | Boolean | false | When true, a progress bar will render on bottom for horizontal and left on vertical. |
progressBarThickness | Number | 4 | Thickness translates to height for horizontal and width for vertical progress bar. |
progressBarBackgroundColor | String | rgba(255,255,255,0.25) | Background color of progress bar background. |
progressBarValueBackgroundColor | String | white | Background color of progress bar value background. |
Prop | Type | Default | Description |
---|---|---|---|
BackgroundComponent | React element | N/A | This component will render in the background of the page and will be animated based on scroll. |
ForegroundComponent | React element | N/A | This component will render in the foreground of the page. |
npm install react-native-parallax-swiper --save
import React from "react"; import { Animated, Text, View, Image, StyleSheet, Dimensions } from "react-native"; import { ParallaxSwiper, ParallaxSwiperPage } from "react-native-parallax-swiper"; const { width, height } = Dimensions.get("window"); export default class App extends React.Component { myCustomAnimatedValue = new Animated.Value(0); getPageTransformStyle = index => ({ transform: [ { scale: this.myCustomAnimatedValue.interpolate({ inputRange: [ (index - 1) * (width + 8), // Add 8 for dividerWidth index * (width + 8), (index + 1) * (width + 8) ], outputRange: [0, 1, 0], extrapolate: "clamp" }) }, { rotate: this.myCustomAnimatedValue.interpolate({ inputRange: [ (index - 1) * (width + 8), index * (width + 8), (index + 1) * (width + 8) ], outputRange: ["180deg", "0deg", "-180deg"], extrapolate: "clamp" }) } ] }); render() { return ( <ParallaxSwiper speed={0.5} animatedValue={this.myCustomAnimatedValue} dividerWidth={8} dividerColor="black" backgroundColor="black" onMomentumScrollEnd={activePageIndex => console.log(activePageIndex)} showProgressBar={true} progressBarBackgroundColor="rgba(0,0,0,0.25)" progressBarValueBackgroundColor="white" > <ParallaxSwiperPage BackgroundComponent={ <Image style={styles.backgroundImage} source={{ uri: "https://goo.gl/wtHtxG" }} /> } ForegroundComponent={ <View style={styles.foregroundTextContainer}> <Animated.Text style={[styles.foregroundText, this.getPageTransformStyle(0)]} > Page 1 </Animated.Text> </View> } /> <ParallaxSwiperPage BackgroundComponent={ <Image style={styles.backgroundImage} source={{ uri: "https://goo.gl/gt4rWa" }} /> } ForegroundComponent={ <View style={styles.foregroundTextContainer}> <Animated.Text style={[styles.foregroundText, this.getPageTransformStyle(1)]} > Page 2 </Animated.Text> </View> } /> <ParallaxSwiperPage BackgroundComponent={ <Image style={styles.backgroundImage} source={{ uri: "https://goo.gl/KAaVXt" }} /> } ForegroundComponent={ <View style={styles.foregroundTextContainer}> <Animated.Text style={[styles.foregroundText, this.getPageTransformStyle(2)]} > Page 3 </Animated.Text> </View> } /> </ParallaxSwiper> ); } } const styles = StyleSheet.create({ backgroundImage: { width, height }, foregroundTextContainer: { flex: 1, alignItems: "center", justifyContent: "center", backgroundColor: "transparent" }, foregroundText: { fontSize: 34, fontWeight: "700", letterSpacing: 0.41, color: "white" } });
Coming Soon…
Quick Links
Legal Stuff