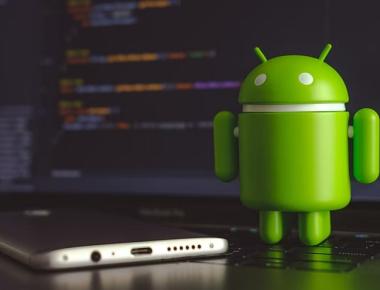
Exploring Alternative Emulators for React Native Development
January 20, 2024
1 min
React Native Radial Menu is a customizable component for creating circular menus in your React Native app. The radial menu consists of a center element and several surrounding elements that are triggered by a press event. The component can be used for a wide range of use cases such as navigation, input selection, and more. Here are some of the props available for the React Native Radial Menu:
onOpen: A callback function that will be called when the radial menu is opened.
onClose: A callback function that will be called when the radial menu is closed.
endAngle: The angle at which the last element of the menu will be positioned, measured in degrees.
radius: The radius of the menu, measured in pixels.
centerItem: The item that will be displayed in the center of the menu.
renderItem: A function that takes an item as a parameter and returns a React element that will be displayed in the menu.
This menu can be fully customized using props.
itemRadius
(Number) 30
- Menu item radiusmenuRadius
(Number) 100
- Distance between root and items in open state.spreadAngle
(Number: 0 - 360) 360
- The angle in degrees based on which menu items are spread on a circle around our root. E.g. 360 full circle, 180 half of circle and so on.startAngle
(Number) 0
- Items are distributed in clockwise direction starting from startAngle. 0 is left, 90 top, and so on.onOpen
(Function) - Called immediately after the menu has entered the open state.onClose
(Function) - Called immediately after the menu has entered the close state.onSelect
(Function) - Called when the item is selectednpm install react-native-radial-menu
import React, { useState } from 'react'; import { StyleSheet, View, Text } from 'react-native'; import RadialMenu from 'react-native-radial-menu'; const App = () => { const [isOpen, setIsOpen] = useState(false); return ( <View style={styles.container}> <RadialMenu onOpen={() => setIsOpen(true)} onClose={() => setIsOpen(false)} distance={70} spacing={35} spreadAngle={150} startAngle={135} isOpen={isOpen} backgroundColor="#fff" mainButton={{ backgroundColor: '#a2a2a2', borderRadius: 20, height: 40, width: 40, justifyContent: 'center', alignItems: 'center', }} mainButtonIcon={ <Text style={{ color: '#fff', fontSize: 24 }}>+</Text> } menuButton={{ backgroundColor: '#a2a2a2', borderRadius: 20, height: 40, width: 40, justifyContent: 'center', alignItems: 'center', }} menuButtonIcon={ <Text style={{ color: '#fff', fontSize: 24 }}>•</Text> } > <View style={styles.menuItem}> <Text style={styles.menuText}>A</Text> </View> <View style={styles.menuItem}> <Text style={styles.menuText}>1</Text> </View> <View style={styles.menuItem}> <Text style={styles.menuText}>2</Text> </View> <View style={styles.menuItem}> <Text style={styles.menuText}>3</Text> </View> <View style={styles.menuItem}> <Text style={styles.menuText}>4</Text> </View> </RadialMenu> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, menuItem: { backgroundColor: '#a2a2a2', borderRadius: 20, height: 40, width: 40, justifyContent: 'center', alignItems: 'center', }, menuText: { color: '#fff', fontSize: 16, fontWeight: 'bold', }, }); export default App;
Coming Soon…
Quick Links
Legal Stuff