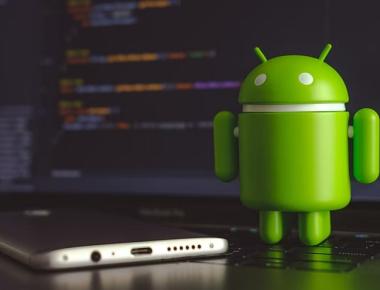
React Native is a popular framework for building native mobile applications using JavaScript and React. However, when it comes to storing data, the framework doesn’t have a built-in solution, which can make it challenging to manage data in a way that is scalable and efficient.
One solution to this problem is to use RxDB, a real-time database that can be used in React Native applications. RxDB provides a number of benefits over traditional databases, including real-time synchronization, offline support, and easy data management.
Getting started with RxDB in React Native is relatively simple. First, you’ll need to install the RxDB library and the required dependencies, including PouchDB and the React Native adapter for RxDB. Once the installation is complete, you can create a new database and define the data schema for the documents you want to store.
In React Native, you can use RxDB to manage data in a variety of ways. For example, you can use it to manage user data, such as user profiles, or to store data about items in your app, such as product information. You can also use RxDB to manage data in real-time, which is especially useful for chat applications.
One of the key benefits of using RxDB in React Native is that it provides real-time synchronization, which means that data changes are automatically reflected on all connected devices in real-time. This makes it easy to keep data up-to-date and consistent across multiple devices.
Another advantage of RxDB is that it provides offline support, meaning that your app can continue to work even when there is no network connection. This makes it ideal for use in situations where internet connectivity may be limited, such as when traveling on an airplane.
Finally, RxDB provides easy data management, allowing you to perform CRUD operations (Create, Read, Update, and Delete) on your data, as well as to perform complex queries and aggregations. This makes it easy to manage and analyze large amounts of data in your React Native app.
In this article, we’ll cover the basics of using RxDB in a React Native project.
To install RxDB, run the following command in your React Native project’s terminal:
npm install rxdb
Then, you can import the rxDB library in your React Native component:
import RxDB from 'rxdb';
Next, you need to define the schema for your database. For example:
javascript
const schema = { version: 0, type: 'object', properties: { name: { type: 'string', }, age: { type: 'number', }, location: { type: 'string', }, }, };
const db = await RxDB.create({ name: 'mydb', adapter: 'memory', }); const collection = await db.collection({ name: 'users', schema: schema, });
await collection.insert({ name: 'John Doe', age: 30, location: 'New York' }); await collection.insert({ name: 'Jane Doe', age: 28, location: 'London' });
6.You can query the collection to retrieve the documents:
const users = await collection.find().exec(); console.log(users);
7.Finally, you can close the database connection when it is no longer needed:
await db.destroy();
This is a basic example of how to use rxDB in a React Native application. You can find more information about the available functions and options in the rxDB documentation.
Quick Links
Legal Stuff