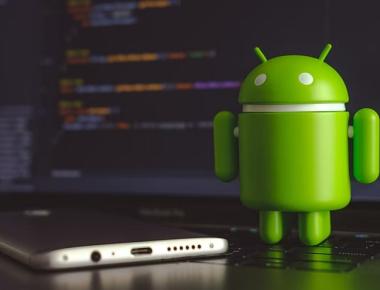
enabled
If false the user won’t be able to interact with the control. Default value is true.
Type | Required |
---|---|
bool | No |
momentary
If true, then selecting a segment won’t persist visually. The onValueChange
callback will still work as expected.
Type | Required | Platform |
---|---|---|
bool | No | iOS |
onChange
Callback that is called when the user taps a segment; passes the event as an argument
Type | Required |
---|---|
function | No |
onValueChange
Callback that is called when the user taps a segment; passes the segment’s value as an argument
Type | Required |
---|---|
function | No |
selectedIndex
The index in props.values
of the segment to be (pre)selected.
Type | Required |
---|---|
number | No |
tintColor
Accent color of the control.
Type | Required |
---|---|
string | No |
backgroundColor
Background color color of the control. (iOS 13+ only)
Type | Required | Supported Version |
---|---|---|
string | No | iOS 13+ |
values
The labels for the control’s segment buttons, in order.
Type | Required |
---|---|
(string | number |
appearance
(iOS 13+ only) Overrides the control’s appearance irrespective of the OS theme
Type | Required | Platform |
---|---|---|
‘dark’, ‘light’ | No | iOS, Android, Web |
fontStyle
(iOS 13+ only) | Type | Required | Platform | | ------ | -------- | -------- | | object | No | iOS, Android, Web |
An object container
color
: color of segment textfontSize
: font-size of segment textfontFamily
: font-family of segment textfontWeight
: font-weight of segment textactiveFontStyle
(iOS 13+ only) | Type | Required | Platform | | ------ | -------- | -------- | | object | No | iOS, Android, Web |
color
: overrides color of selected segment textfontSize
: overrides font-size of selected segment textfontFamily
: overrides font-family of selected segment textfontWeight
: overrides font-weight of selected segment texttabStyle
(Android and Web only) Styles the clickable surface which is responsible to change tabs | Type | Required | Platform | | ------ | -------- | -------- | | object | No | Android, Web |
npm install react-native-segment-control --save --force
import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; import SegmentControl from 'react-native-segment-control'; const One = () => { return <Text style={styles.text}>This is first view</Text>; }; const Two = () => { return <Text style={styles.text}>This is second view</Text>; }; const Three= () => { return <Text style={styles.text}>This is 3rd view</Text>; }; const Four = () => { return <Text style={styles.text}>This is 4th view</Text>; }; const segments = [ { title: 'One', view: One }, { title: 'Two', view: Two }, { title: 'Three', view: Three }, { title: 'Four', view: Four } ]; const App = () => { return ( <View style={styles.container}> <SegmentControl segments={segments} /> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#F5F7FA', justifyContent: 'center' }, text: { alignSelf: 'center', margin: 50 } }); export default App;
Quick Links
Legal Stuff