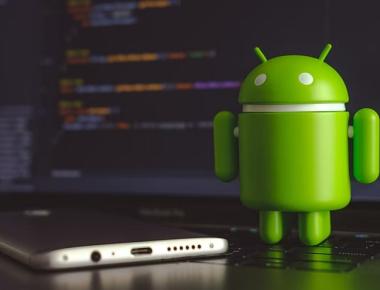
React Native is a popular platform for developing cross-platform mobile applications. One of the key features of mobile applications is the ability to use the various sensors available on a device to enhance the user experience. In this article, we will discuss the different sensors that can be used in React Native and how to use them in your applications.
The accelerometer is a sensor that measures the acceleration and tilt of a device. It can be used to detect the orientation of the device, determine if the device is being shaken, and even track the user’s step count. To use the accelerometer in React Native, you can use the react-native-sensors library. This library provides a simple API for accessing the accelerometer data and using it in your applications.
The gyroscope is a sensor that measures the angular velocity of a device. It can be used to determine the orientation of the device and detect any changes in orientation. To use the gyroscope in React Native, you can use the react-native-sensors library in the same way as you would use the accelerometer.
The proximity sensor is a sensor that detects the presence of an object near the device. It is commonly used to turn off the screen when a user brings the device close to their face during a call. To use the proximity sensor in React Native, you can use the react-native-proximity library. This library provides a simple API for accessing the proximity data and using it in your applications.
The magnetometer is a sensor that measures the magnetic field of a device. It can be used to determine the direction in which the device is facing, and can also be used for compass applications. To use the magnetometer in React Native, you can use the react-native-sensors library in the same way as you would use the accelerometer and gyroscope.
Light Sensor
The light sensor is a sensor that measures the ambient light level in the environment. It can be used to automatically adjust the brightness of the device’s screen based on the ambient light level. To use the light sensor in React Native, you can use the react-native-light-sensor library. This library provides a simple API for accessing the light data and using it in your applications.
To get started, you will need to install the react-native-sensors library. You can do this using the following command:
npm install react-native-sensors
or
yarn add react-native-sensors
Once the library has been installed and linked, you can start using it in your React Native applications. The react-native-sensors library provides a simple API for accessing the sensors on a device. To use the sensors, you will need to import the react-native-sensors library in your code, and then use the Accelerometer or Gyroscope classes to access the data from the sensors.
Here is an example of how to use the Accelerometer class in your code:
import { Accelerometer } from "react-native-sensors"; const accelerometer = new Accelerometer({ updateInterval: 400 // defaults to 100ms }); accelerometer.subscribe(({ x, y, z }) => { console.log("Accelerometer:", x, y, z); });
In this example, we import the Accelerometer class from the react-native-sensors library, and then create an instance of the Accelerometer class. The updateInterval property is set to 400ms, which means that the accelerometer data will be updated every 400ms.
We then subscribe to the accelerometer object, and in the callback function, we log the accelerometer data to the console. The data is provided as an object with x, y, and z properties, which represent the acceleration along the x, y, and z axes, respectively.
Similarly, you can use the Gyroscope class to access the data from the gyroscope sensor:
import { Gyroscope } from "react-native-sensors"; const gyroscope = new Gyroscope({ updateInterval: 400 // defaults to 100ms }); gyroscope.subscribe(({ x, y, z }) => { console.log("Gyroscope:", x, y, z); });
In this example, we import the Gyroscope class from the react-native-sensors library, and then create an instance of the Gyroscope class. The usage is similar to the Accelerometer class, with the only difference being the type of sensor that is being accessed.
By using these sensors, you can enhance the user experience and create more interactive and engaging applications. Whether you are developing a fitness app that uses the accelerometer to track steps, or a compass app that uses the magnetometer to determine the direction, the sensors available in React Native provide a wide range of possibilities for creating exciting and innovative mobile applications.
Quick Links
Legal Stuff